Data structures are fundamental building blocks for efficient software systems. As BCA students, you will need to learn data structures to organise and manage information. You might think of DS as a toolbox to hold various tools for specific tasks. When you need them, you retrieve them and use them.
In this BCA study guide, you will learn various applications of Data Structures – from searching the web to implementing algorithms. We will start with the basics of three essential data structures:
- Queues,
- Stacks, and
- Hash Tables.
Queues
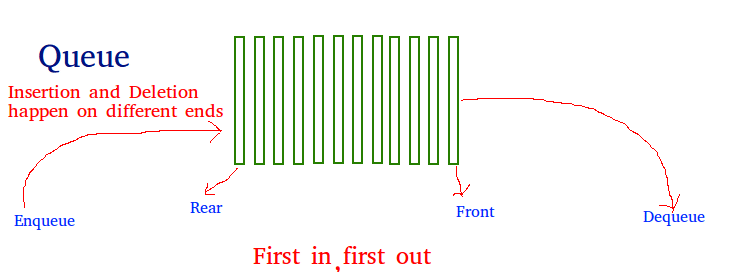
Imagine a queue at a cash counter in a mall. People join the line at the back and everyone is served on a ‘first-in, first-out’ (FIFO) basis. Similarly, in data structures, a queue follows the same principle. New elements are added at the ‘end’ of a queue and when elements are retrieved, those that are added first get retrieved first.
Some applications of ‘Queues’ in Data Structures are:
- Task scheduling: Operating systems often use queues to manage processes waiting for CPU time.
- Breadth-first search algorithms: In graph theory, exploring neighbouring nodes level by level often involves using queues.
As BCA students, you might have observed queues at work in:
1. Printer spooler: When you send a document to print, it doesn’t directly go to the printer. Instead, it is added to a queue managed by the operating system’s spooler service. The spooler acts like a queue, holding multiple print jobs and processing them one after another based on their order in the queue.
2. Music playlist: Music streaming services or media players often allow users to create playlists. These playlists function like queues. Songs are added in the order you choose, and they are played one after the other, maintaining the order they were added.
3. Download managers: When you download multiple files from the internet, a download manager typically queues them. This ensures downloads happen sequentially, preventing overloading the internet connection and potential delays.
Since this is a BCA study guide, we will suggest a few project ideas where you can use ‘queues’ in data structures:
1. Call Centre Management System: It can use ‘queues’ for incoming calls. Calls can wait based on their position in the queue until a representative is available to assist them.
2. Customer Order Processing System: It can use ‘queues’ for managing incoming orders. These orders can be processed sequentially – in the order they come in – to ensure fairness in the system and that no orders are missed.
Stacks
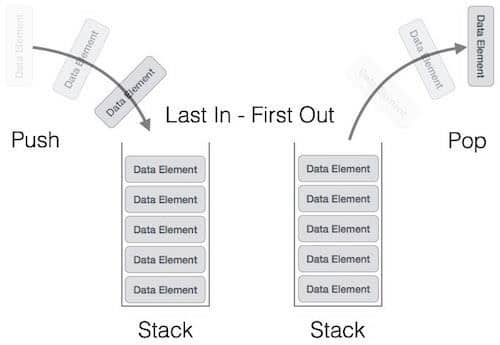
Stacks are essentially the opposite of queues. Think of a stack of books. The last book is added to the top of the stack – and when you have to remove it, you will pick it first (since it’s at the top). So, it uses the ‘last-in, first-out’ (LIFO) principle. Elements are added (or pushed) onto the top of the stack and removed (popped) from the top as well.
There are two main applications of stacks BCA students will see:
- Function call stack: When a program calls a function, information about the function call is pushed onto a stack. When the function returns, the information is popped off.
- Undo/redo functionality: In many software programs, stacks are used to implement undo and redo features. This allows users to reverse actions.
BCA students might have seen stacks at work in:
- Browser history, where web browsers use stacks to keep track of web pages users have visited. Users can navigate through them in ‘History’ pushing and popping elements to and from the stack.
- Data conversion, when stacks are used to convert digits using different number bases (e.g., decimal to binary) and perform operations based on the target base.
A few ideas for Data Structures projects where BCA students use stacks can be:
1. Infix to Postfix Conversion: This program converts an infix arithmetic expression (e.g., “(A + B) * C”) to a postfix expression (e.g., “A B + C *”) will use stacks to process the expression. It will push operands (numbers) onto the stack and handle operators according to their precedence. Then, it will pop elements from the stack to construct the postfix expression.
2. Balanced Parenthesis Checker: This program checks whether a given string contains balanced parentheses (round, square, curly). here, opening brackets (e.g., (, {, [) are pushed onto the stack and when a closing bracket is encountered, the top element from the stack is popped to compare with the closing bracket. If they don’t match or the stack becomes empty prematurely, the expression is considered unbalanced.
3. Simple Calculator: You might create a basic calculator program that can perform arithmetic operations like addition, subtraction, multiplication, and division. The stack stores operands (numbers) entered by the user. You pop two operands from the stack when an operator is encountered, perform the operation, and push the result back onto the stack.
4. Maze Solver: This program can solve a maze represented as a 2D grid. You can use the stack to keep track of the path taken during the exploration. If a dead end is reached, pop the last move and explore an alternative path. Backtracking utilizes the stack to revisit previous options.
Hash Tables
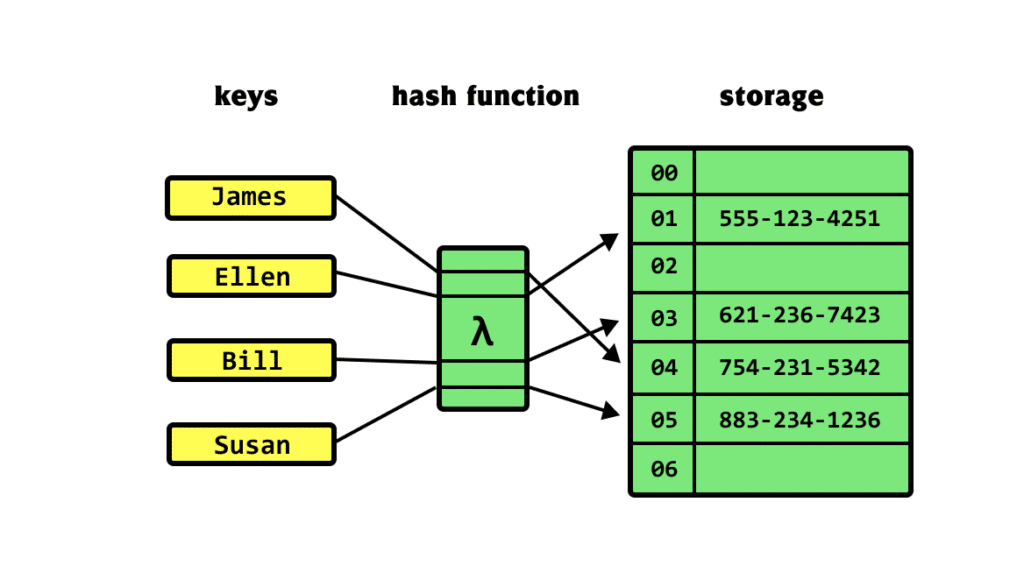
This BCA study guide hopes to make it easy to imagine how data can be structured in different ways using real-world examples. Hash tables are like books arranged in a library according to different categories. If you know the book’s category code, you can retrieve a specific book much faster.
Hash tables operate in a similar fashion. These tables store key-value pairs. A hash function is used to convert a key (like a book title) into a unique code (like a category code). This code is called a hash and is used to determine the location of the table where the book (or the value) is stored.
The reason we use hash tables is that it makes searches much faster. Each hash has a fixed number of elements and the average search time within a hash is constant. So, if you have a very large dataset and you know the hash where the value can be stored, the retrieval becomes much more efficient. For applications like symbol tables that compilers use, hash tables are ideal for retrieving specific data.
As BCA students, you might have seen hash tables at work in:
1. Caching Mechanisms: Operating systems and web browsers often use hash tables for caching frequently accessed data. When you request the same file or webpage again, the data acts as the key and you see it much faster as it is retrieved from the hash table (cache) instead of the original source.
2. In-Memory Databases: Modern in-memory databases often use hash tables for storing and retrieving data to look up things faster. Each data record is assigned a key based on a specific field and based on the query, this key is used to retrieve individual records efficiently.
3. Network Routing: In routing tables in networks, the destination IP address acts as the key and the value stores information like the next hop router on the path. Hash tables enable efficient routing decisions by quickly determining the path for data packets based on their destination addresses.
Wondering if this BCA study guide is going to offer you data structure project ideas that use hash tables? Well, here are some for you:
1. Simple Dictionary App: This program will function like a basic dictionary. Users can search for word definitions using the word itself as the key. The hash table can store word-definition pairs. The hash function can be based on the first few letters of the word. Students can practise key-value lookups and understand collision handling mechanisms.
2. Duplicate File Finder: Scanning a directory to identify duplicate files will require a hash table to store the unique hash values of each file’s content. The program will calculate the hash value and check if it already exists in the hash table. If the same hash value is found, the program can report the file name.
3. Basic Data Management: A simple in-memory database that explores the concept of associative arrays can use hash tables to manage data and add, retrieve, and delete key-value pairs using the respective functionalities. Here, students can practise CRUD (Create, Read, Update, Delete) operations using hash tables.
4. Spell Checker with Auto-Correction: You can even create a basic spell-checker program of your own. The program will check for misspelt words and suggest corrections. One hash table will store correctly spelt words while the other one will store potential misspelt words with suggested corrections.
Whenever a user types in a word, the program first compares it with correctly spelt words. If it’s not there, it will compare it with misspelt words and suggest corrections. In this project, students will also have to use some string manipulation techniques for text processing.
Remember, this is just the beginning of Data Structures. BCA students will go on to study advanced structures like binary trees, heaps, and graphs which we will cover later in our other article.
Keep following the blog to learn more.